목록JavaScript (37)
Mi Lugarcito
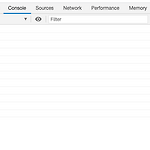
class Counter{//클래스 (다양한 오브젝트를 만들기 위해서) constructor(runEveryFiveTimes){//클래스가 처음 생성되면 this.counter=0;//counter라는 변수가 있는데 0부터 시작한다 this.callback = runEveryFiveTimes; } //클래스 안에 함수가 있다.(class에서 함수 선언할때는 function 이라고 표시 안해도 된다) increase(){ this.counter++; console.log(this.counter); if(this.counter % 5===0){ this.callback(this.counter);//5배수일때마다 콜백 } } } function printSomething(num){ console.log(`wo..
//함수도 object 에 포함된다! 함수의 reference 가 메모리에 만들어진다 function add (num1, num2){ return num1+num2; } function devide(num1, num2){ return num1/num2; } function surprise(operator){//실행 const result=operator(2,3);//할당 === //add(2,3)이랑 동일한 효과 & devide(2,3)이랑 동일한 효과 console.log(result); } surprise(devide); //함수의 이름을 다른 변수에 할당해도 레퍼런스는 같다. // const doSomething = add; // const result = doSomething(2,3); // co..
//number, string, boolean, null, undefined //number, string, boolean, null, undefined, symbol 타입을 제외한 모든것들은 object라고 한다. //object : 최소 한두가지 데이터들을 한군데에 모아둔것을 말한다.(배열, 리스트...함수...등) //number, string, boolean, null, undefined (데이터 단위가 작아서 메모리에 그대로 들어온다) //number, string, boolean, null, undefined, symbol 타입을 제외한 모든것들은 object라고 한다. //object : 최소 한두가지 데이터들을 한군데에 모아둔것을 말한다.(배열, 리스트...함수...등) let number..
//함수 선언-1 function doSomething(add){ console.log(add); const result = add(1,2); console.log(result); } //함수 선언-2 function add(a,b){ const sum = a+b; return sum; } //함수 호출-1 //doSomething(add);//실행하면 add라는 함수 자체가 호출되어 나타난다 (add()이렇게가 아니고 @@) //함수 호출-2 // const result = add(1,2); // console.log(result); const addFun = add; console.log(add); addFun(1,2);
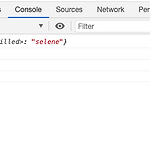
www.notion.so/07dfed016e914c3a8612fc76dd1542f0?v=c6feaeb5b46e4fdeb1e756113cb529c1 드림코딩 by 엘리 JS 강의 정리 사이트 링크 → https://www.youtube.com/watch?v=wcsVjmHrUQg&list=PLv2d7VI9OotTVOL4QmPfvJWPJvkmv6h-2&index=2 www.notion.so promise 복습 milugarcito.tistory.com/408 async & await : promise보다 좀 더 간결하게 !! 동기적으로 실행되는 것 처럼 보이게 해준다. (실은 비동기이다) 1. async => promise를 사용하지 않아도 함수앞에 async 붙여주기만 하면 {}안에 들어있는 코드 블럭들이 ..
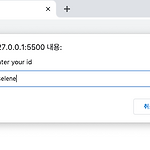
'use strict'; //Promise is a JavaScript object for asynchronous operation //비동기적 수행시 callback 함수 대신 유용하게 쓸 수 있는 object //State : pending -> fulfilled or rejected //Producer vs Consumer //1. Producer //promise는 class이다. new 키워드를 통해 obj 만들 수 있다. //promise를 만드는 순간 execute 라는 callback 함수 (여기서는 resolve)가 바로 실행된다. // when new Promise is created, the executor runs automatically.... const promise = new ..
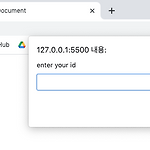
synchronous : 정해진 순서에 맞게 코드가 실행되는 것 asynchronous : 비동기적으로 언제 코드기 실행될지 예측할 수 없는것 callback 함수 ? 전달해준 함수를 나중에 함수 호출해주는 것 (setTimeout 같은 경우 callback 함수의 api) 'use strict'; //JavaScript is synchronous (동기적) => 정해진 코드 순서에 맞추어 순서대로 코드 실행되는것 // Execute the code block by orger after hoisting (hoisting 된 이후부터 나타나는 코드가 순서에 맞춰 하나씩 동기적 실행) // hoisting ? var변수 또는 function declaration 함수 선언들이 자동으로 제일 위로 올라가는것 ..
Object Serialize : 직렬화 해서 json으로 변환 deserialize : object로 다시 변환하는것 overloading : 함수의 이름은 동일하지만 어떤 종류의 파라미터를 (전달되는 매개변수) 전달하느냐, 몇개의 파라미터를 전달하느냐에 따라서 각각 다른 방식으로 호출이 가능하다 //Json //JavaScript Object Notation //1. Object to JSON //stringify(obj) let json = JSON.stringify(true); console.log(json); json=JSON.stringify(['apple', 'banana']); console.log(json);//["apple","banana"] 배열타입처럼 보여진다 //object 정의 ..
배열함수 10가지 필수 (join, split, reverse, splice, enrolled, filter, map, some, reduce(right), sort) //아주 중요 : 배열 함수 10가지 //Q1. make a string out of an array(배열을 스트링으로 변환하기) { const fruits =['apple', 'banana', 'orange']; const result = fruits.join(','); console.log(result);//apple,banana,orange } //Q2. make an array out of a string (string을 배열로 만들기) { const fruits = '🍅, 🥝,🍌,🍑 '; const result = fruits.s..
'use strict'; //Array //1. Declaration const arr1 = new Array(); const arr2 = [1,2]; //2. Index position const fruits = ['🍏', '🍌']; console.log(fruits); console.log(fruits.length); console.log(fruits[0]); console.log(fruits[1]); console.log(fruits[fruits.length-1]);//마지막 데이터 찾기 //3. Looping over an array 무한루프 돌리기 //print all fruits console.clear(); //a. for for(let i=0; i console.log(fruit)); //..